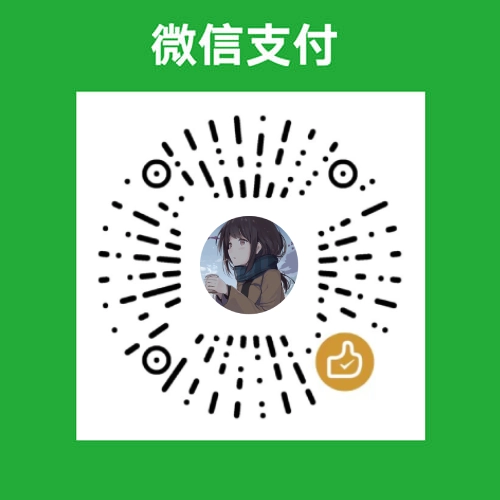
Next.js 搭建官网踩坑小记
依赖版本说明
Next.js
13tailwindcss
3.xpostcss
8.xantd
4.x
配置模块路径别名
Next.js
自 9.4
起自动支持 tsconfig.json
和 jsconfig.json
中的 "paths"
和 "baseUrl"
选项,因此可以不在 webpack
配置项中配置模块路径别名
新建 tsconfig.json
或者 jsconfig.json
文件
项目不使用
TypeScript
时使用jsconfig.json
进行配置
json
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"@/*": ["src/*"]
}
}
}
使用 webpack 进行配置
修改 next.config.js
文件
js
const path = require('path')
/** @type {import('next').NextConfig} */
const nextConfig = {
webpack: (config) => {
config.resolve.alias['@'] = path.resolve(__dirname, 'src')
return config
}
}
module.exports = nextConfig
Absolute Imports and Module Path Aliases | Next.js
使用 tailwindcss
sh
# 安装依赖
npm i -D tailwindcss postcss autoprefixer
# 生成配置文件
npx tailwindcss init -p
Install Tailwind CSS with Next.js
使用 antd 4.x
sh
# 安装依赖
npm i antd@4 @ant-design/icons@4
自定义主题
方案一:使用 Variable 方案
修改 src/pages/_app.tsx
文件
diff
+ import 'antd/dist/antd.variable.min.css'
import '@/styles/globals.css'
import type { AppProps } from 'next/app'
import { ConfigProvider } from 'antd'
+ ConfigProvider.config({
+ theme: {
+ primaryColor: '#abcdef'
+ }
+ })
export default function App({ Component, pageProps }: AppProps) {
return <Component {...pageProps} />
}
方案二:使用 Less 方案
安装依赖
sh
npm i next-plugin-antd-less
npm i -D babel-plugin-import
添加 .babelrc.js
文件
js
module.exports = {
presets: [['next/babel']],
plugins: [['import', { libraryName: 'antd', style: true }]]
}
修改 next.config.js
文件
js
const withAntdLess = require('next-plugin-antd-less')
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
swcMinify: true
}
module.exports = withAntdLess({
...nextConfig,
modifyVars: {
'@primary-color': '#abcdef'
}
})
导航栏高亮选中
封装一个组件使用 cloneElement
对 children
进行拦截处理
配置 Layout
修改 src/pages/_app.tsx
配置默认 Layout
js
import Header from '@/layout/Header'
import Footer from '@/layout/Footer'
export default function App({ Component, pageProps }: AppPropsWithLayout) {
const getLayout =
Component.getLayout ??
((page) => (
<section>
<Header />
{page}
<Footer />
</section>
))
return getLayout(<Component {...pageProps} />)
}
在需要自定义 Layout
的页面添加 getLayout
方法
js
const Login = () => <div>登录页</div>
Login.getLayout = (page) => page
export default Login
在 Next.js 13
中提供了一种新的自定义 Layout
的方法 Migrating the getLayout() pattern to Layouts (Optional)
静态 HTML 导出
静态 HTML
导出不需要 Node.js
服务,即可独立运行,适用于页面内容在构建时就能确定的场景(静态官网、文档网站等)
修改 package.json
json
{
"scripts": {
"export": "next build && next export"
}
}
其他配置项
修改启动端口
修改 package.json
json
{
"scripts": {
"dev": "next dev -p 4000"
}
}
相关路径配置
js
const isDevelopment = process.env.NODE_ENV === 'development'
/** @type {import('next').NextConfig} */
const nextConfig = {
/* 构建输出目录 */
distDir: isDevelopment ? '.next_dev' : '.next',
/* 静态资源前缀 */
assetPrefix: isDevelopment ? undefined : './',
/* 应用基础路径 */
basePath: ''
}
module.exports = nextConfig